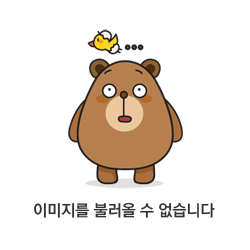
Factory Method란?
Factory Method는 객체 생성을 캡슐화하여 객체 생성 로직을 클래스의 하위 클래스 또는 인터페이스를 통해 책임을 이전하는 디자인 패턴입니다. 즉, 객체 생성을 호출하는 코드와 실제 객체 생성 로직을 구현하는 코드를 분리하여 유지보수성과 확장성을 개선하는 패턴입니다.
이 패턴에서는 객체를 생성하는 메서드를 인터페이스로 정의하고, 이를 구체적인 클래스에서 구현합니다. 이렇게 함으로써 객체 생성을 추상화하고, 객체 생성을 변경해야 할 경우 인터페이스를 수정하는 것으로 대체할 수 있습니다.
Class Diagram(UML)
패턴에 대한 전체적인 구조와 관계를 파악하려면 Class Diagram을 살펴보는 게 좋습니다. Factory Method패턴에 대한 클래스다이어그램(Class Diagram)은 아래와 같습니다.
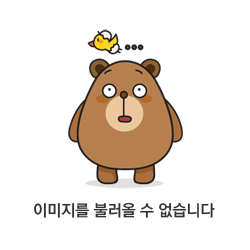
- Creator : 객체를 생성하는 메서드를 선언하는 추상 클래스 또는 인터페이스
- ConcreteCreator : "Creator" 클래스를 상속받아 "ConcreteProduct" 객체를 생성하는 메서드를 구현한 클래스
- Product : 생성될 객체에 대한 추상 클래스 또는 인터페이스
- ConcreteProduct : "Product" 추상 클래스 또는 인터페이스를 상속받아 구현한 구체적인 클래스
적용 예제
먼저 구현하려는 Factory Method에 대한 클래스 다이어 그램을 예로 살펴보겠습니다.(아래 클래스 다이어 그램은 C++ 기준으로 작성되었습니다.)
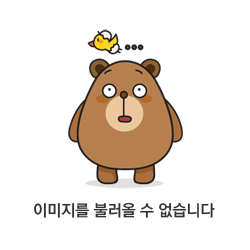
위 클래스 다이어 그램에서 Animal과 AnimalFactory는 추상 클래스나 인터페이스를 나타냅니다. Dog와 Cat은 Animal 클래스를 상속받아 구현한 클래스이고, DogFactory와 CatFactory는 AnimalFactory를 상속받아 구현한 클래스입니다.
AnimalFactory 클래스는 CreateAnimal() 추상 메서드를 선언하고, DogFactory와 CatFactory 클래스는 이 메서드를 구현하여 Dog와 Cat 객체를 생성합니다. AnimalFactory 클래스는 객체를 생성하는 데 필요한 데이터, 예를 들어 animalType 등의 정보를 가질 수도 있습니다. CreateAnimal() 메서드는 Animal 클래스의 포인터를 반환합니다.
다음은 C++로 Factory Method를 구현한 예제입니다.
#include <iostream>
using namespace std;
// Animal 클래스
class Animal {
public:
virtual void Speak() = 0;
};
// Dog 클래스
class Dog : public Animal {
public:
void Speak() {
cout << "멍!멍!" << endl;
}
};
// Cat 클래스
class Cat : public Animal {
public:
void Speak() {
cout << "야~옹!" << endl;
}
};
// AnimalFactory 인터페이스
class AnimalFactory {
public:
virtual Animal* CreateAnimal() = 0;
};
// DogFactory 클래스
class DogFactory : public AnimalFactory {
public:
Animal* CreateAnimal() {
return new Dog();
}
};
// CatFactory 클래스
class CatFactory : public AnimalFactory {
public:
Animal* CreateAnimal() {
return new Cat();
}
};
int main() {
AnimalFactory* factory;
Animal* animal;
// DogFactory를 이용하여 Dog 객체 생성
factory = new DogFactory();
animal = factory->CreateAnimal();
animal->Speak();
// CatFactory를 이용하여 Cat 객체 생성
factory = new CatFactory();
animal = factory->CreateAnimal();
animal->Speak();
return 0;
}
결과
멍!멍!
야~옹!
다음은 C#으로 Factory Method 예제를 동일하게 구현하였습니다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DesignPatternExam
{
// Animal 클래스
abstract class Animal
{
public abstract void Speak();
}
// Dog 클래스
class Dog : Animal
{
public override void Speak()
{
Console.WriteLine("멍!멍!");
}
}
// Cat 클래스
class Cat : Animal
{
public override void Speak()
{
Console.WriteLine("야~옹!");
}
}
// AnimalFactory 인터페이스
interface IAnimalFactory
{
Animal CreateAnimal();
}
// DogFactory 클래스
class DogFactory : IAnimalFactory
{
public Animal CreateAnimal()
{
return new Dog();
}
}
// CatFactory 클래스
class CatFactory : IAnimalFactory
{
public Animal CreateAnimal()
{
return new Cat();
}
}
class Program
{
static void Main(string[] args)
{
IAnimalFactory factory;
Animal animal;
// DogFactory를 이용하여 Dog 객체 생성
factory = new DogFactory();
animal = factory.CreateAnimal();
animal.Speak();
// CatFactory를 이용하여 Cat 객체 생성
factory = new CatFactory();
animal = factory.CreateAnimal();
animal.Speak();
}
}
}
결과
멍!멍!
야~옹!
댓글